Read and Write JSON in Python
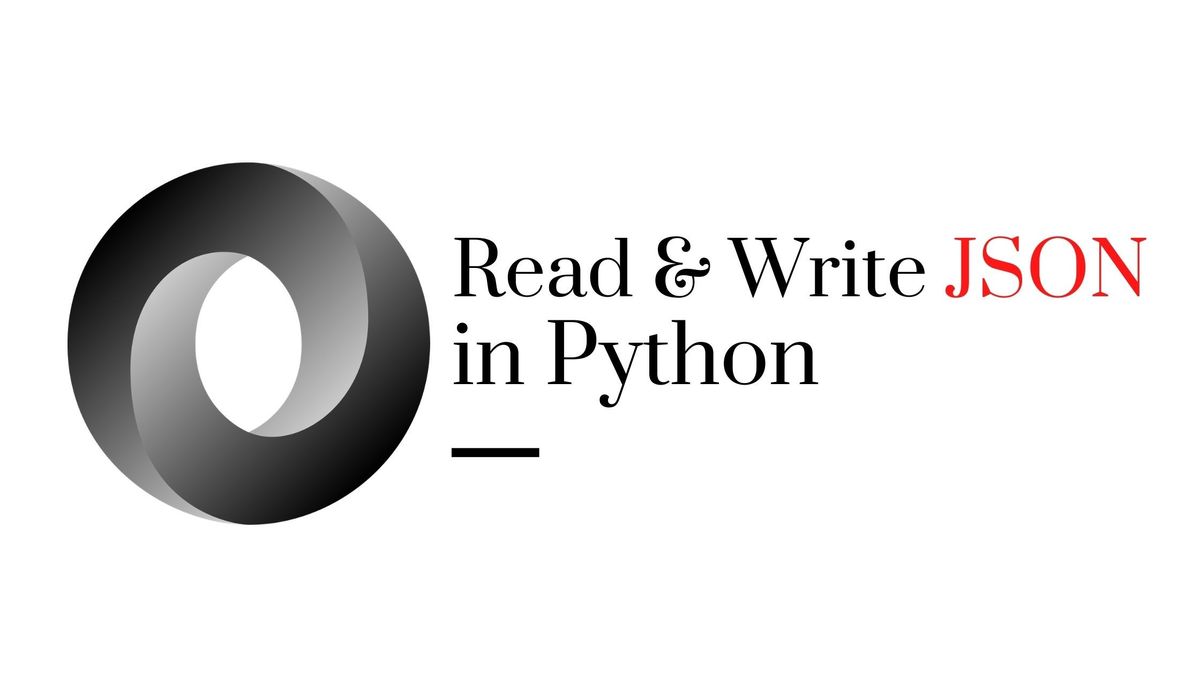
What is a JSON file?
According to Wikipedia JSON is “JavaScript Object Notation (JSON, pronounced /ˈdʒeɪsən/; also /ˈdʒeɪˌsɒn/) is an open standard file format, and data interchange format, that uses human-readable text to store and transmit data objects consisting of attribute–value pairs and array data types (or any other serializable value).”
In Short JSON is:
- It is Light-weight file format to exchange data.
- Data is written in key : value pairs. just like dictionaries in python.
Read JSON
Luckily Python has an in-built package named ‘JSON’ which can parse and manipulate data.
import json #imports json package
To parse the json file we need open the json file in python and then pass the file object in json.load() function.
JSON file :
import json
f=open("summary.json",'r')
#open json as file object
data=json.load(f) #parse file object
print(data)
#print parsed json
Next, we want to access the key: vale pairs in the json file.
Just like dictionaries in python, data can be accessed by using indexing where ‘key’ is index value.Remember Keys are case sensitive.
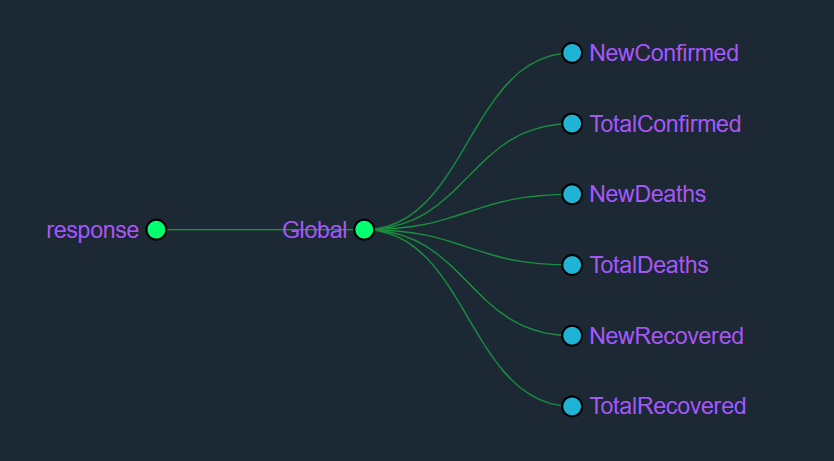
import json
f=open("summary.json",'r')
#covid-19 data 16aug2020
data=json.load(f)
print(data['Global']) #print value in 'Global' key.
print(data['Global']['NewConfirmed']) #print integer value in new confirmed
f.close()
Write JSON
As we have already seen that JSON is very much similar to python dictionaries therefore the simplest way to create a JSON file would be to convert dictionary into JSON.
Json dump function does the job of json creation easily.
import json
data={
"person":{
"name":"Rajesh",
"age":"23",
"gender":"M",
"height":"5.5ft"
},
}
#make a dictionary with key-value pairs
with open('datadump.json', 'w') as json_file: #open file to write
json.dump(data, json_file) #dump json data