Read File in Python
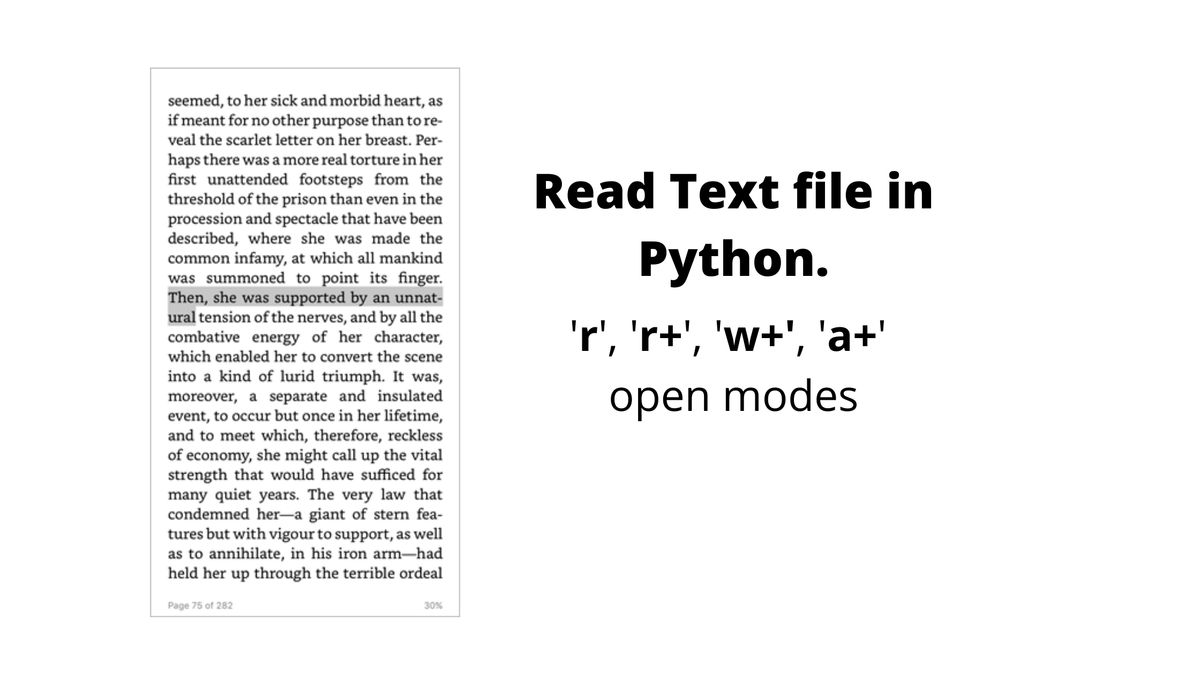
To read a txt file in python “read” function is used, but before this we have to open the file in “read mode”.
To open the file in read mode ‘r’ argument is used in the open function.
Demo Code to read file in python
f = open("demofile.txt", "r")
data= f.read()
print(data)
The Above code open the file “demofile.txt” in ‘r’ mode (read) then the read function read the full content of the file.
Optional: Argument ‘size’ of read function reads only limited bytes of text.
- read(10) will read only 10 bytes of data from the file
- read(-1) will read the full text in file acts similar to read()
How to Read line by line?
‘readlines’ function returns a list containing lines from the file. ‘\n’ which is escape character for ‘newline’ denotes the end of the line.
Demo Code for readlines
f = open("demofile2.txt", "r")
data=f.readlines()
print(data)
‘readlines’ returns a list which is saved in the data variable. You can print each line individually by iterating over the list and accessing each element.
Bonus:
Addition to the majorly used :
- ‘r’ read mode
- ‘w’ write mode
- ‘a’ append mode
Open also supports:
- ‘r+’ read and write, moves the cursor at the start
- ‘w+’ write and read, cleans the file(truncate) and then write
- ‘a+’ append and read, moves the cursor at the end

This flowchart is so far the best explanation to modes of open file in python.
You might also like : How to Write to File in Python.
Flowchart credit: https://stackoverflow.com/questions/1466000/difference-between-modes-a-a-w-w-and-r-in-built-in-open-function/30566011#30566011